- Dev Random Linux In Command
- Dev Random Linux In C 10
- Dev Random Linux
- Dev Random Linux In C Download
Using cat to read from /dev/urandom is a bad idea, because it will try to read /dev/urandom to the end - but it does not end. You can use head. But take care to read by byte, not by line - because lines would be randomly separated by random newline bytes. So, to read 30 random bytes into a file random.bytes, use: head -c 30 /dev/urandom. I'm looking for ways to use /dev/random (or /dev/urandom) from the command line.In particular, I'd like to know how to use such a stream as stdin to write streams of random numbers to stdout (one number per line). I'm interested in random numbers for all the numeric types that. Oct 02, 2019 Contribute to torvalds/linux development by creating an account on GitHub. Linux kernel source tree. Contribute to torvalds/linux development by creating an account on GitHub. The /dev/random driver under Linux uses minor numbers 8 and 9 of. the /dev/mem major number (#1). So if your system does not have. /dev/random and /dev/urandom. Entropyavail does not indicate the number of bits available in /dev/random.It indicates the kernel's entropy estimate in the RNG state that powers /dev/random.That entropy estimate is a pretty meaningless quantity, mathematically speaking; but Linux blocks /dev/random if the entropy estimate is too low.
P: n/a | In the following piece of code, which simply generates a sequence of (random) octal codes, I'm surprised by the apparent non-randomness of /dev/random. It's not noticeable unless RAND_LENGTH is largish. I was under the assumption that /dev/random was 'more random' than /dev/urandom, and that it would block if it ran out of entropy until it got more. Why am I seeing so many zeroes in my output? #include <stdio.h> #include <fcntl.h> #define RAND_LEN 1024 void read_random( const char* dev ) { int i, fd; char dat[RAND_LEN]; fd = open( dev, O_RDONLY ); dat[RAND_LEN] = '0'; if( fd != -1 ) { read( fd, dat, RAND_LEN ); for( i = 0; i < RAND_LEN; i++ ) { dat[i] = (dat[i] >> 4 & 0x07) + 48; } printf( '%s: %snn', dev, dat ); } else { exit( 1 ); } close( fd ); } int main( void ) { read_random( '/dev/random' ); read_random( '/dev/urandom' ); return( 0 ); } 506$ ./test /dev/random: 20005061706352643760251034665507247403176742630660 50462223206040017306150002434274350703035033674561 20754173510400040000000050000000000007737204577300 00177356040504450022043404000034041773610464046404 00000000000000000000000000000000000000000000000000 00000000000000000000000000640423461000000000000000 00000000000000000000000000002404000000004100700000 00634610000000000000000000000000000000000000000000 00000000000000000000000000005604000000000000340437 73040037730504300000004773000000000000000000000000 00003000100000000000000000000000000000000000000000 00000000000000000000000000000450045004000000002000 70041304330407040000170434041704050427730704050400 30160457732404000067040000000000000000000070042000 64040000340400006404677300045004000000005773200070 04130433040704000017043404640440004773070440000770 00007773240400005704000000000000000000000000000034 04710007047773220417046704000000000000777300000000 00000000077007735404050416046304070457733773020470 00200040044773577311046414641437736504641457733404 000054041773220464045704 /dev/urandom: 10313700276075133047145322754731744715330540305544 55772411741125544374737071744665152471472301655103 21673032612147044376361336471103425426601777564215 27537506506760532046755677545002343626131545501130 60140067534031123241466461750705230751645572440071 57744425536470274352332415164131435115125573101442 74556544717777054520152351764241666207571007700122 35623563012311717741547124617262165454244161061517 74201651063200446176135471662402411424412532245563 11545142476223664702172000553652612247511550424514 72612104741103553240132614710710775524572432011176 15642610621436346764640170271720054525337367306022 20367455521635765367573615753123615162510360064030 26113642124742630634422243216772330177360713406462 47534536645354770361405340770217451441530701353725 67322557767603402406511316575637667506305617702430 35222250564070154307150656704155003661646277201332 46523045641760343566266442731667313365016666076430 23673210310073545356647564170524251663066260134503 70701275067537603265673560312322526077022157223404 211323047413633213326054 -- Ron Peterson Network & Systems Manager Mount Holyoke College
|
|
- C Standard Library Resources

Description
The C library function int rand(void) returns a pseudo-random number in the range of 0 to RAND_MAX.
RAND_MAX is a constant whose default value may vary between implementations but it is granted to be at least 32767.
Declaration
Time of your life david cook mp3 free download. Following is the declaration for rand() function.
Parameters
Dev Random Linux In Command
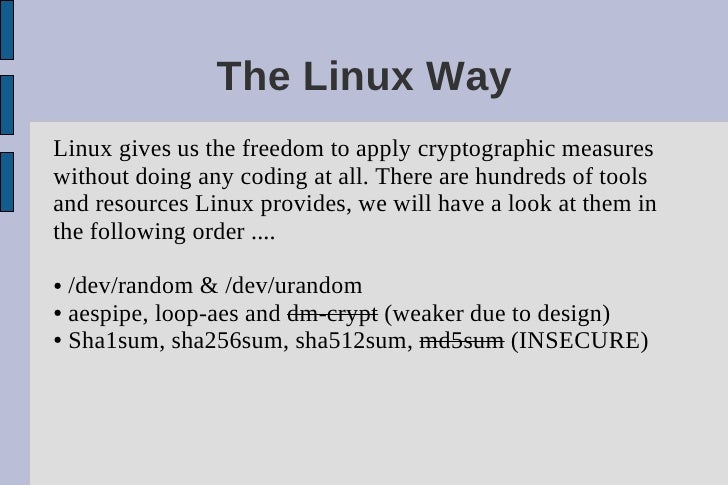
Return Value
This function returns an integer value between 0 and RAND_MAX.
Dev Random Linux In C 10
Example
The following example shows the usage of rand() function.
Dev Random Linux
Let us compile and run the above program that will produce the following result −
Dev Random Linux In C Download
stdlib_h.htm